Templates in Pack: Understanding and Managing Page Structures
Templates are only available on Pack storefronts.
Changing page's section template
You can change a page section template inside the Customizer:
- Navigate to the Customizer.
- Click on Section Template settings in the left panel.
- Select a template from the dropdown menu.
- Click Save.
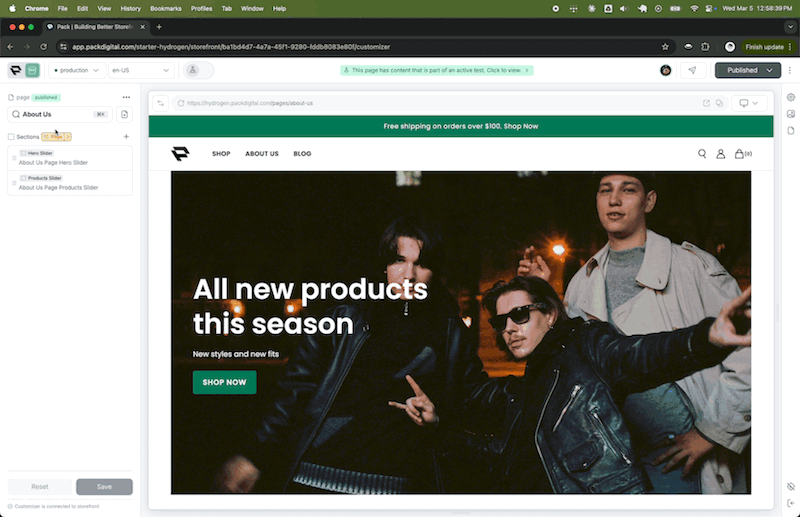
Creating a section template
You can create a new template in Pack’s admin:
- Navigate to Pack’s admin.
- Click on Sections > Templates in the left sidebar.
- Click on Create template in the top right corner.
- Enter a name for your template, select a template type and optionally add sections.
- Click Save.
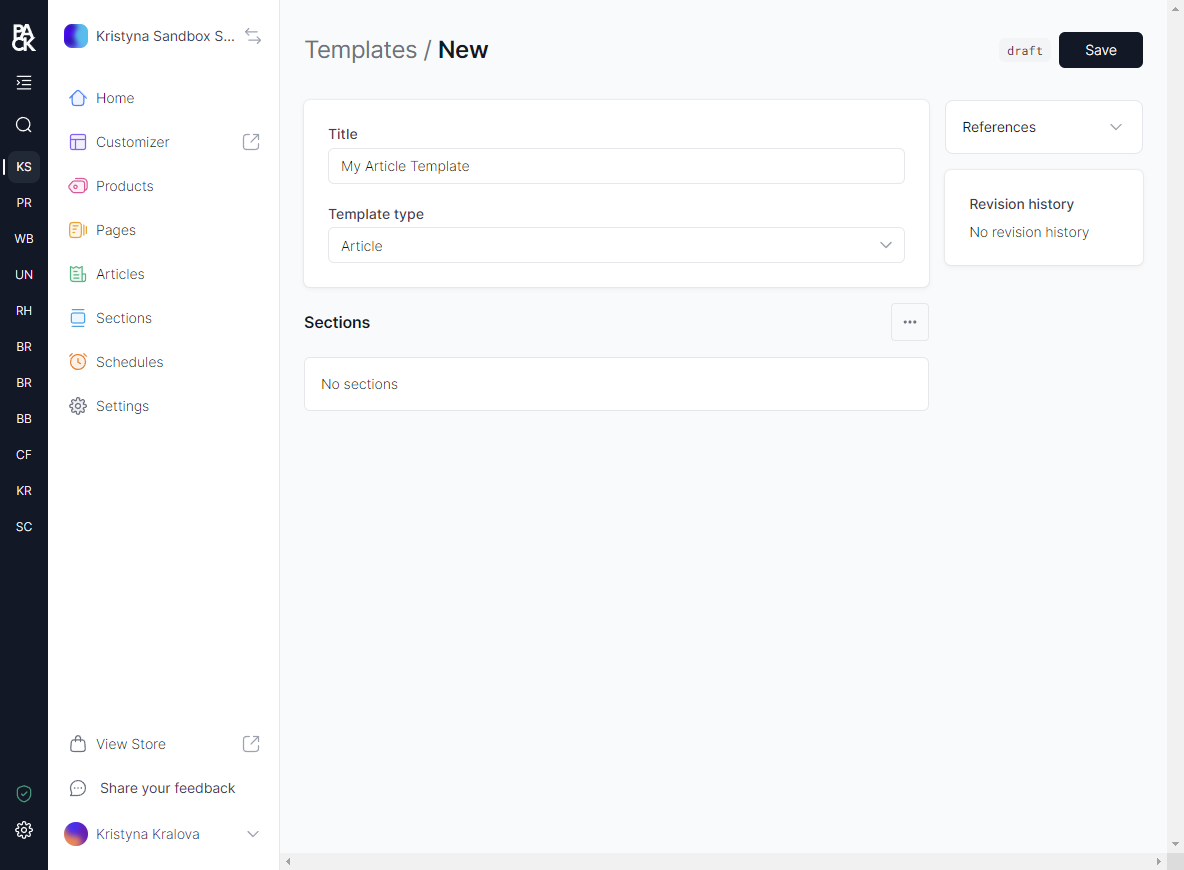
Editing a section template
You can edit a section template in Pack’s admin:
- Navigate to Pack’s admin.
- Click on Sections > Templates in the left sidebar.
- Click on the template you want to edit.
Duplicating a section template
You can easily duplicate a template with all its sections in Pack’s admin:
- Navigate to Pack’s admin.
- Click on Sections > Templates in the left sidebar.
- Click on the three dots next to the template you want to duplicate.
- Choose whether you want to copy the existing sections as new sections or link to the existing sections.
Deleting a section template
Currently, you cannot delete a template. This feature is coming soon.
Publishing a section template
You can publish a template in Pack’s admin:
- Navigate to Pack’s admin.
- Click on Sections > Templates in the left sidebar.
- Click on the three dots next to the template you want to publish.
- Click Publish.
You can follow the same steps to unpublish a template.
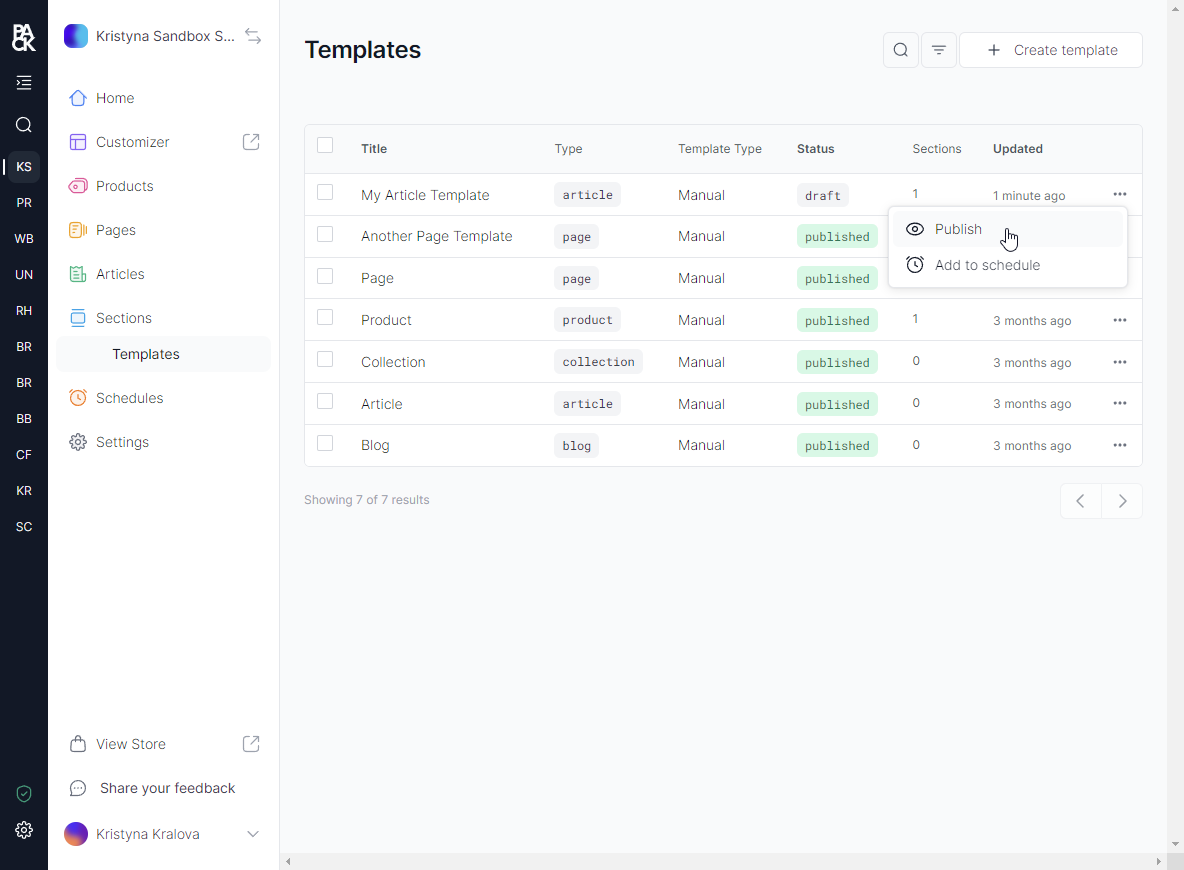