Blueprint Theme Architecture: Components and Customization
Pack’s Blueprint is like an advanced, component-based Hydrogen theme that’s designed to help you spin up a Shopify Hydrogen storefront quickly.
We’ve enhanced Shopify Hydrogen's out-of-the-box demo site, providing a rich, customizable, storefront that is ready to deploy.
Access Pack’s Blueprint for storefronts on Github.
Access Pack’s Blueprint for shops on Github.
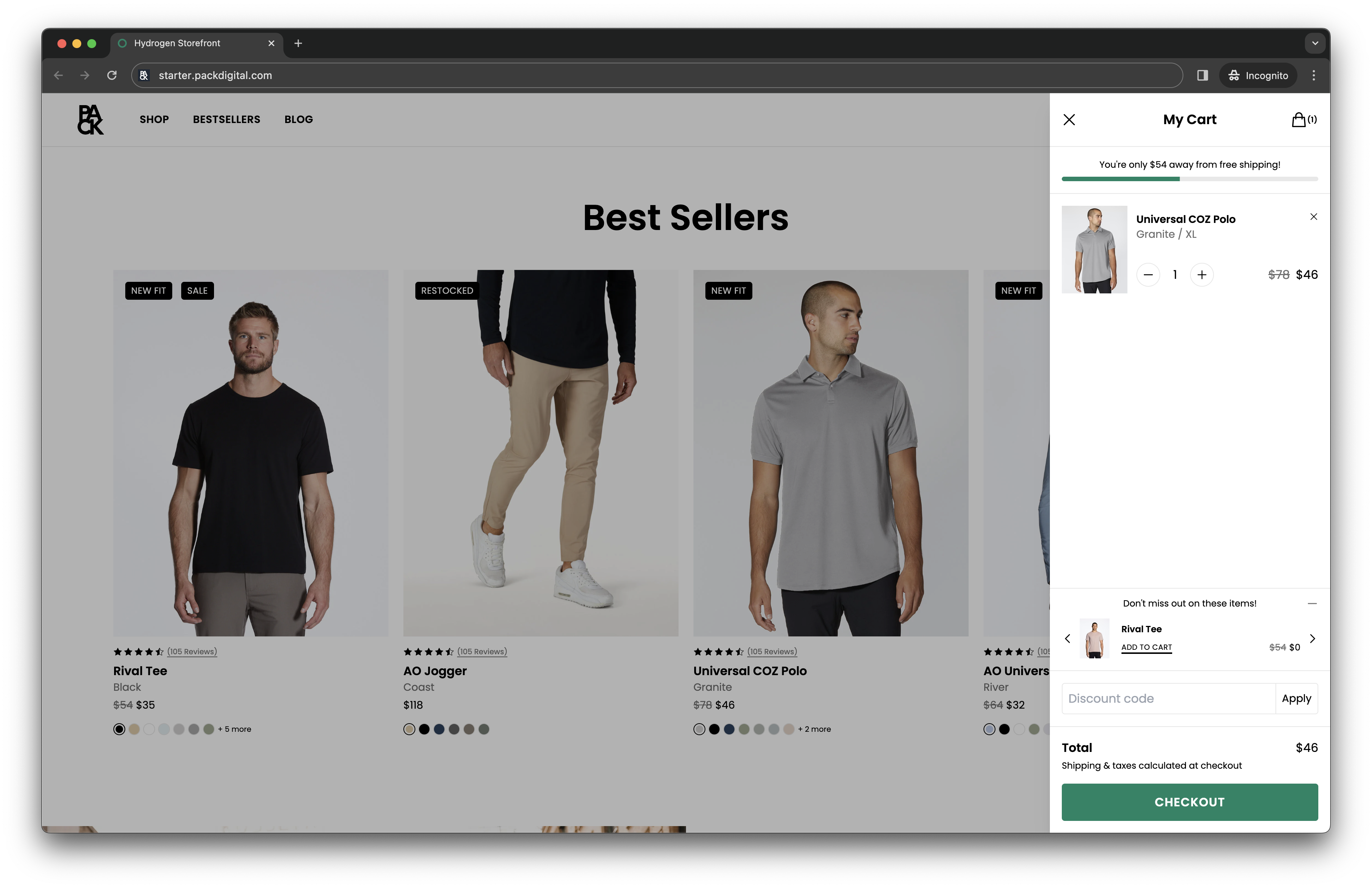
Key benefits:
- Enhanced user interactions: subtle animations throughout elevate the user experience
- Integrated with Pack’s Sections and Schemas: Offers a variety of functionalities including hero sections, tile grids, and testimonial sliders, enabling you to create a store that caters to your unique needs.
- Data and analytics: Data layer logic through GA4
- Advanced Cart Functionality: Introduces enriched cart features such as a free shipping meter and upsell options, elevating the shopping experience.
- Supports product grouping: Use Pack’s product grouping feature to create groupings or bundles you can use in upsell experiences.
Technical Specs
Remix: By leveraging Remix for server-side rendering, Pack’s Blueprint Theme gives you high control over how assets load, as well as faster page loads and performance.
Tailwind CSS: adds a layer of responsiveness and visual appeal, guaranteeing a seamless experience across all devices. Plus, we’ve done some light styling and animations to give it all a bit more polish.
Typescript: Helps minimize runtime errors, easy to strip out if you prefer Javascript.
Cart API: Built using Shopify’s cart API.
Features at a Glance:
Responsive components
- Shoppable Social Video
- Heros—multiple slides and configurations images
- 50/50 Heros
- Tile rows
- Tile grids
- Product tiles
- Image tiles
- Text/markdown blocks
- Video blocks
- Video embed blocks
- Testimonials slider
- Product slider
- Reviews slider
- Form Builder
- Accordions
- Icon Rows
Customize any of these components, or create new ones and add them to Pack’s component library. Then add components from the library to any page in Pack’s customizer, edit the, (copy, imagery etc.), and push it live—no technical background required.
Site settings, menus and modals
- Promo bar
- Modal
- Mobile sidebar menu
- Footer
- Email newsletter sign up
- Country selector (change currency, UI/UX for language change)
- Header
- Dropdown menus
- Slide out mobile menu
Use the global settings schema to customize the site’s look and feel, and add new settings as needed.
Search, data & analytics
- Search sidebar
- Search page
- Leverages Shopify’s API
- SEO and schema markup fields
Side cart
- Free shipping meter
- Cart upsell product slider
- Discount code field
Shopify analytics
- Page views
- Add to carts
Data layer logic through GA4 (QA’d by Elevar + Fueled)
Triggers placed throughout the site:
- Login
- Register
- Add to cart
- Remove from cart
- View cart
- PDP view
- Collection view
- Product item click
- Email Subscribe
- Phone subscribe
- Search results
Automatic frontend product groupings
Based on groupings set in Pack admin.
Getting Started
Implementing Pack's Blueprint Theme involves several key steps, including server configuration adjustments and leveraging loaders for dynamic content. For a detailed walkthrough, refer to the available guides and documentation resources.
To hydrogen-themedive deeper into integrating Pack's Hydrogen Theme, explore our detailed integration guide.